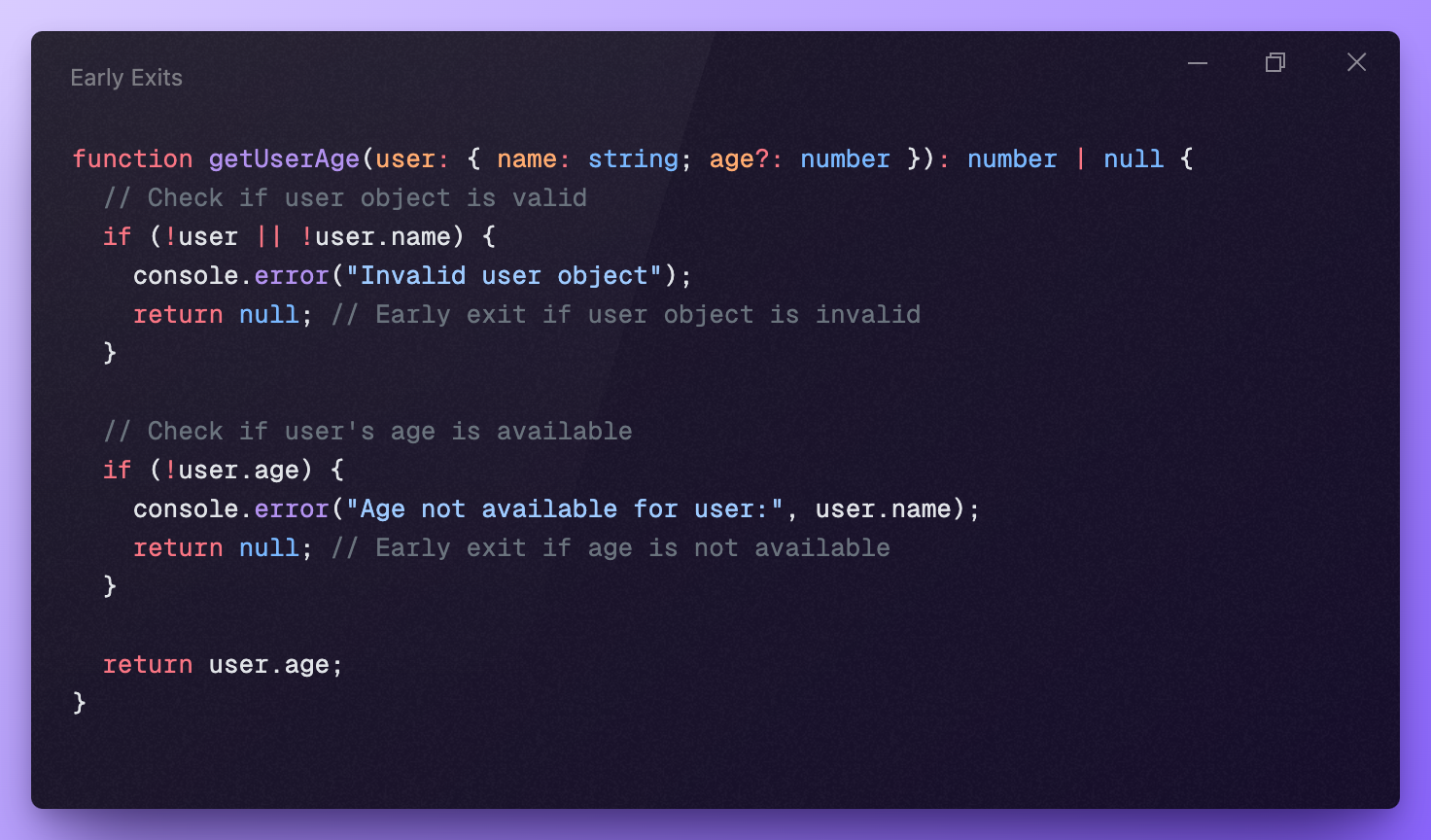
Early Exits
What are Early Exits?
Early Exits, as the name suggests, are all about making a quick exit from a function or block of code if certain conditions are met. Instead of nesting your code under numerous if-else statements or letting it cascade down into a deep pyramid of indentation, Early Exits allow you to gracefully bow out if a particular condition isn’t met, simplifying your code and making it more readable.
Let’s Break It Down with an Example
Imagine you’re writing a function to fetch the age of a user from an object containing their name and age. Here’s a snippet of what that function might look like in TypeScript:
function getUserAge(user: { name: string; age?: number }): number | null {
// Check if user object is valid
if (!user || !user.name) {
console.error("Invalid user object");
return null; // Early exit if user object is invalid
}
// Check if user's age is available
if (!user.age) {
console.error("Age not available for user:", user.name);
return null; // Early exit if age is not available
}
return user.age;
}
// Example usage:
const user1 = { name: "Alice", age: 30 };
const user2 = { name: "Bob" };
const user3 = null;
console.log(getUserAge(user1)); // Output: 30
console.log(getUserAge(user2)); // Output: Age not available for user: Bob, null
console.log(getUserAge(user3)); // Output: Invalid user object, null
So, What’s Happening Here?
In this example, we’re using Early Exits to streamline our function and make it more robust.
First, we check if the user object exists and if it contains a name. If not, we log an error and exit early, preventing further execution of the function. Then, we check if the age property is available in the user object. If it’s missing, we log another error and exit early once again.
#The Benefits of Early Exits Now, you might be wondering, why bother with Early Exits when you could just write a bunch of nested if-else statements and achieve the same result? Well, here’s why:
-
Readability: Early Exits make your code cleaner and easier to follow. By handling exceptional cases first, the main flow of your code becomes more apparent, reducing cognitive load for both you and anyone else who might read your code.
-
Error Handling: By exiting early and handling exceptional cases upfront, you’re less likely to encounter unexpected errors later in your code. This proactive approach to error handling can save you countless headaches down the road.
-
Performance: Early Exits can also lead to performance improvements, especially in functions with complex logic. By exiting early when conditions aren’t met, you avoid unnecessary computations, resulting in faster execution times.
So, there you have it—Early Exits in a nutshell. Next time you find yourself writing code, remember the power of making a quick exit when conditions aren’t right. Your future self—and anyone who has to maintain your code—will thank you for it! Happy coding! 🚀👩💻