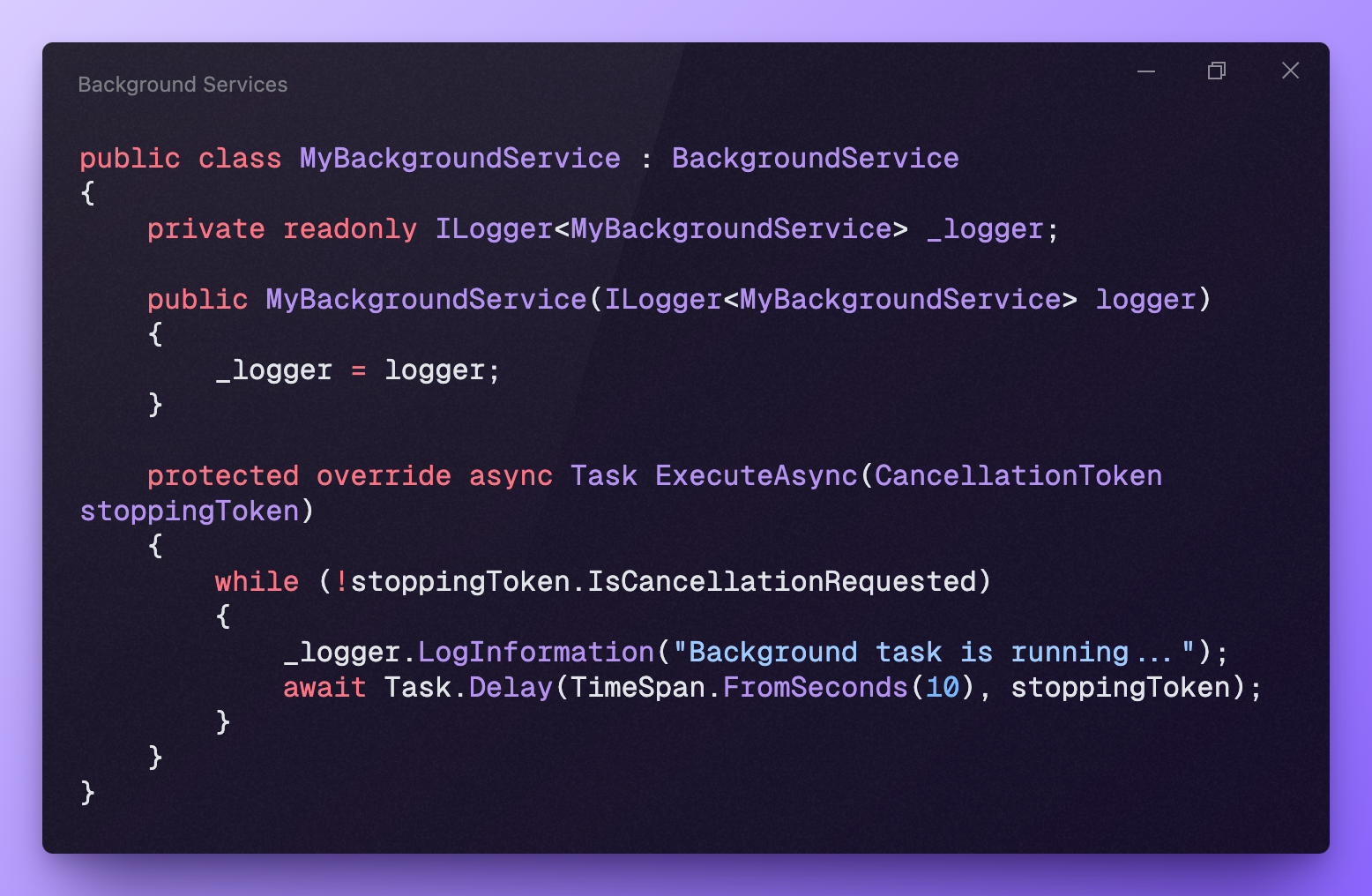
Demystifying Background Tasks in .NET: A Practical Guide
Hey, fellow developers! Today, we’re diving into a topic that’s essential for building robust and efficient applications in .NET: background tasks.
Understanding Background Service vs Hosted Service
When it comes to background tasks in .NET, two terms often come up: background service and hosted service. Let’s break down the differences between them:
Background Service
A background service is a long-running task that runs independently in the background of your application. In ASP.NET Core, background services are typically implemented by creating a class that inherits from the BackgroundService base class. These services are managed by the ASP.NET Core dependency injection system and can perform tasks like processing messages from a queue, performing periodic cleanup tasks, or updating cached data.
Example Usages
Let’s take a look at a simple example of a background service that performs a task at regular intervals:
public class MyBackgroundService : BackgroundService
{
private readonly ILogger<MyBackgroundService> _logger;
public MyBackgroundService(ILogger<MyBackgroundService> logger)
{
_logger = logger;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
_logger.LogInformation("Background task is running...");
await Task.Delay(TimeSpan.FromSeconds(10), stoppingToken);
}
}
}
In this example, MyBackgroundService inherits from BackgroundService and implements the ExecuteAsync method to define the task to be performed. The service logs a message every 10 seconds until cancellation is requested.
Hosted Service
A hosted service, on the other hand, is a more generic concept that can be used in various types of .NET applications, not just ASP.NET Core. It’s defined by implementing the IHostedService interface, which requires the implementation of the StartAsync and StopAsync methods. Hosted services are responsible for starting and stopping background tasks or services within the application’s lifetime.
Background Service: A Singleton Experience
One important aspect to note about background services in .NET is that they are singleton instances by default. This means that there’s only one instance of the service running throughout the lifetime of your application. While this can be advantageous for managing shared state or resources, it’s essential to be mindful of potential concurrency issues and ensure that your background tasks are thread-safe.
Leveraging IServiceProvider for Dependency Injection
In .NET applications, dependency injection (DI) is a powerful pattern that allows you to decouple components and promote code reuse and testability. When working with background tasks, you often need to access other services within your application. Thankfully, .NET provides a convenient way to do this using the IServiceProvider interface.
The IServiceProvider interface represents a container for resolving services. You can use it to retrieve instances of required services by calling the GetServiceanti-pattern
, however in this case, it is the only way to access instances of services we need.
Due to this, BackgroundServices can become a bit messy and we should remember that we should still handle them like normal services, which includes all the patterns we use for writing clean code.
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using System;
using System.Threading.Tasks;
public class MyBackgroundService : BackgroundService
{
private readonly ILogger<MyBackgroundService> _logger;
private readonly IServiceProvider _serviceProvider;
public MyBackgroundService(ILogger<MyBackgroundService> logger, IServiceProvider serviceProvider)
{
_logger = logger;
_serviceProvider = serviceProvider;
}
protected override async Task ExecuteAsync(Task stoppingToken)
{
using (var scope = _serviceProvider.CreateScope())
{
var myService = scope.ServiceProvider.GetRequiredService<MyService>();
// Use myService here...
}
try
{
while (!stoppingToken.IsCompleted)
{
_logger.LogInformation("Background task is running...");
await Task.Delay(TimeSpan.FromSeconds(10)); // Delay for 10 seconds
}
}
catch (OperationCanceledException)
{
// Handle cancellation gracefully if needed
_logger.LogInformation("Background task has been cancelled.");
}
}
}
Harnessing the Power of CancellationToken
CancellationToken is a versatile tool for managing asynchronous operations in .NET. It allows you to signal cancellation to a task, giving it the opportunity to clean up resources and gracefully terminate. When working with background tasks, it’s essential to use CancellationToken to handle cancellation requests gracefully.
You can pass a CancellationToken to your background tasks and periodically check for cancellation using the IsCancellationRequested property. By doing so, you can ensure that your tasks respond to cancellation requests in a timely manner and release any resources they may be holding.
public class MyBackgroundService : BackgroundService
{
private readonly ILogger<MyBackgroundService> _logger;
private CancellationToken _cancellationToken;
public MyBackgroundService(ILogger<MyBackgroundService> logger)
{
_logger = logger;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
_cancellationToken = stoppingToken;
try
{
while (!_cancellationToken.IsCancellationRequested)
{
_logger.LogInformation("Background task is running...");
// Simulate some work being done
await Task.Delay(TimeSpan.FromSeconds(10));
}
}
catch (OperationCanceledException)
{
// Handle cancellation gracefully if needed
_logger.LogInformation("Background task has been cancelled.");
}
}
public void StopBackgroundService()
{
_cancellationToken.ThrowIfCancellationRequested();
}
}
Exploring Alternatives like Hangfire and Others
While .NET provides built-in support for background tasks with background services, you may also explore third-party libraries for more advanced job scheduling and background processing features. Let’s take a look at some popular alternatives:
Hangfire
Hangfire is a powerful background processing library for .NET that allows you to perform background processing, scheduling, and recurring tasks with ease. It provides a simple yet robust API for managing background jobs, enabling features like delayed jobs, recurring tasks, and distributed processing.
Features of Hangfire:
- Recurring Jobs: Schedule jobs to run at specified intervals.
- Delayed Jobs: Execute jobs after a certain delay.
- Dashboard: Monitor and manage background jobs through a web-based dashboard.
- Fault Tolerance: Handle failures gracefully with automatic retries and error handling.
- Distributed Processing: Scale your background processing across multiple servers or processes.
Example Usage of Hangfire:
// Configure Hangfire in Startup.cs
services.AddHangfire(config => config.UseSqlServerStorage(connectionString));
// Enqueue a background job
BackgroundJob.Enqueue(() => Console.WriteLine("Hello, Hangfire!"));
Quartz.NET
Quartz.NET is a feature-rich scheduling library for .NET applications. It provides powerful scheduling capabilities, including support for cron expressions, job persistence, and clustering.
Features of Quartz.NET:
- Cron Expressions: Schedule jobs using flexible cron expressions.
- Job Persistence: Store job and trigger information in a database for resilience.
- Clustering: Scale your application across multiple nodes with built-in clustering support.
- Listeners and Triggers: Customize job behavior with listeners and triggers.
Example Usage of Quartz.NET:
// Configure Quartz.NET scheduler
ISchedulerFactory schedulerFactory = new StdSchedulerFactory();
IScheduler scheduler = await schedulerFactory.GetScheduler();
// Define and schedule a job
IJobDetail job = JobBuilder.Create<MyJob>().Build();
ITrigger trigger = TriggerBuilder.Create()
.WithIdentity("myTrigger")
.WithCronSchedule("0 0/1 * 1/1 * ? *") // Run every minute
.Build();
await scheduler.ScheduleJob(job, trigger);
await scheduler.Start();
Azure Functions
Azure Functions is a serverless compute service that enables you to run event-driven code without managing infrastructure. It’s well-suited for running background tasks, processing messages from queues, and responding to events.
Features of Azure Functions:
- Serverless: Pay only for the resources you consume, with automatic scaling and management.
- Event-Driven: Trigger functions in response to events from Azure services or custom triggers.
- Integration: Seamlessly integrate with other Azure services like Azure Storage, Azure Service Bus, and Azure Cosmos DB.
- Bindings: Simplify input and output handling with built-in bindings for Azure services.
Example Usage of Azure Functions:
// Define an Azure Function to process queue messages
public static class QueueProcessor
{
[FunctionName("QueueProcessor")]
public static async Task Run(
[QueueTrigger("myqueue-items", Connection = "AzureWebJobsStorage")] string myQueueItem,
ILogger log)
{
log.LogInformation($"C# Queue trigger function processed: {myQueueItem}");
}
}
Comparison
Feature | Hangfire | Quartz.NET | Azure Functions |
---|---|---|---|
Job Scheduling | ✔️ | ✔️ | ✔️ |
Delayed Jobs | ✔️ | ✔️ | ❌ |
Recurring Tasks | ✔️ | ✔️ | ❌ |
Distributed | ✔️ | ✔️ | ✔️ |
Dashboard | ✔️ | ❌ | ❌ |
Serverless | ❌ | ❌ | ✔️ |
Cost | Self-hosted | Self-hosted | Pay-per-use |
When choosing a background processing solution, consider factors such as the complexity of your scheduling requirements, scalability needs, and cost considerations. Hangfire and Quartz.NET are excellent choices for on-premises or self-hosted scenarios, while Azure Functions provides a serverless alternative with seamless integration into the Azure ecosystem.
Conclusion
And there you have it—background tasks demystified! By understanding the differences between background services and hosted services, leveraging the power of dependency injection with IServiceProvider, and embracing CancellationToken for graceful task management, you’ll be well-equipped to build efficient and responsive applications in .NET. Whether you stick with the built-in background service functionality or explore alternatives like Hangfire, the key is to choose the approach that best fits your application’s requirements. Happy coding! 🌟👨💻